Like most of my fun little side projects, the Discord bot started with the question of not whether I should, but whether I could. It was an academic exercise in the growing field of “Hey, can I put an AI chatbot into this thing?” Which, of course, for Discord, the answer is an obvious, “Well, duh.”
I’ve started using Discord lately. My plan is to initiate a weekly chat among anyone interested in working on nondescript “projects.” While those quotes may sound suspicious, I assure you everything is “above board.”
Since I was already there, I figured it was the perfect time and place to create something. Specifically, something with LangChain, a technology I’ve been using, learning, and deeply fascinated by.
What Is LangChain?
You may be familiar with Large Language Models (LLMs) — what we now so affectionately refer to as “Artificial Intelligence” or AI. Unlike some naysayers, I embrace the term AI for these tools. They pass the criteria for the Turing Test, the classic measure of a machine’s intelligence. In short, that test is whether or not a computer can convince another human that they are indeed human (Some humans struggle passing this test). The goalposts on that are probably moving as I speak.
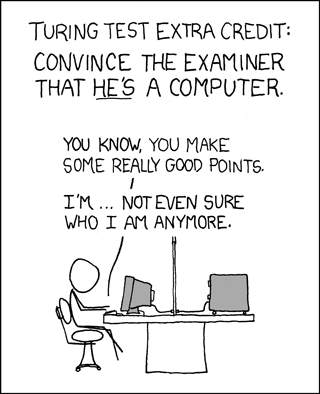
LangChain is a framework that enables you to incorporate LLMs into workflows, or “chains” of actions. It simplifies much of the boilerplate required to write AI tools from scratch. For even more complex workflows, there’s LangGraph, which might be considered its superior. As the names imply, LangChain is built with a chain of processes in mind, where LangGraph is built for a graph of interconnected nodes of processes.
LangChain supports nearly every LLM you can think of, including OpenAI’s ChatGPT, Claude, and my personal favorite service, Together AI. It even works with local models via Ollama for you weirdos who like to run your own models locally (like me). This flexibility allows you to use multiple models with custom prompts in a single workflow.
LangChain also supports saving embeddings, enabling models to remember context via databases like Postgres or Pinecone.
It can also read documents or websites, enabling Retrieval-Augmented Generation (RAG). In simpler terms, it retrieves documents and feeds them into the LLM’s context for analysis.
Additionally, LangChain facilitates tool use, where the LLM produces structured outputs that can trigger applications, scripts, or functions with parameters parsed and decided by the LLM. These features combine to create what are called “AI Agents” — Programs infused with AI models capable of executing complex tasks with some degree of agency.
Applying These Concepts to a Discord Bot
Let’s break down the technical concepts into a practical example: creating a Discord bot.
- Basic Replies: You can plug an LLM like ChatGPT into a Discord app to reply to chat messages. A system prompt could give the bot a distinct personality.
- RAG: The bot could use Discord chat history as its data source. By incorporating chat data into the LangChain workflow, the bot could “read” conversations and answer questions about them.
- Tool Use: The bot could perform actions based on commands. For example, if an admin says, “Bot, ban TurboNoodleGiggletron9000 please…(sigh)…again,” the LLM could generate data that triggers LangChain to issue a
/ban
command for the specified user ID.
With these components, you’d have an AI Agent acting as a Discord bot. At its highest aspiration, it could function as a Discord moderator.
Building Surlybot
I wrote Surlybot in Python. The code itself is surprisingly simple. In fact, getting the permissions to make the bot private and installable on my server was more challenging than writing the bot.

Here’s some notes and the code.
- LLM Integration: I used LangChain to import GPT-4o-mini from OpenAI.
- Discord Library: I utilized
discord.py
for handling interactions. For now, the bot only responds to requests with$robot
, but this can easily be expanded with additional triggers. (note: all of the documentation to set up an app/bot in discord are detailed in the discord.py documentation) - System Prompt: The
SystemMessage()
function in LangChain defines the bot’s personality as a “rude and surly” robot. - Authentication: The
client.run
function requires a bot key from the Discord application control panel. Also you need an Open AI key which you can get from Open AI settings. I’ve commented mine out because I have an environment variable of OPENAI_API_KEY set for my API key which LangChain automatically uses for Open AI auth.
# This example requires the 'message_content' intent.
import logging
import discord
import os
from langchain_openai import ChatOpenAI
from langchain_core.messages import HumanMessage, SystemMessage
# os.environ["OPENAI_API_KEY"] = "Your open ai key here"
llModel = ChatOpenAI(model="gpt-4o-mini")
intents = discord.Intents.default()
intents.message_content = True
client = discord.Client(intents=intents)
@client.event
async def on_ready():
print(f'We have logged in as {client.user}')
@client.event
async def on_message(message):
if message.author == client.user:
return
if message.content.startswith('$robot'):
messages = [
SystemMessage("Pretend you are a rude and surly robot who only gives begrudging responses."),
HumanMessage(message.content),
]
logging.info(message.content)
llmResponse = llModel.invoke(messages)
await message.channel.send(llmResponse.content)
client.run('MTMzMTQ1NzM4NTE1OTQ1ODg3Ng.GwELkH.9jO2fZPJKsiAbajJON8sQzAFN-c9JxMyKL6xYY')

Next Steps
This bot is still in its infancy. Some next goals include:
- Hosting: Moving the bot to the cloud so I don’t need to keep it running on my local machine.
- Extending Functionality: Adding more capabilities and exploring how LangChain can enhance its interactions.
- Building Interfaces: Developing a proper interface between Discord and LangChain to streamline the workflow.
Surlybot is a fun, practical way to experiment with LLMs and LangChain. Whether it’s being a rude chatbot or a capable moderator, the possibilities are endless. If you’re into AI and Discord, I highly recommend giving this a try.
[…] once was a robot named SurlyBot. I hope you’re not expecting this to become a limerick. Because nothing rhymes with […]